Code of Internship Portal Project in PHP
The Internship Portal project helps the Internee of the university to search their internship easily. The system has two users the Administrator and Internees. The Administrator users add, delete, view, update and search/maintain the data of the system such as the Internship application. The Administrator is in charge of populating the list of Internship Job Availability. The Internee user can explore/search their desired internship job and can send their application. Any Internees have the privilege to apply for the posted interns but only those who are registered can apply for the post.
The project was build using PHP, HTML 5, Bootstrap, CSS, JS, and MySQL Database. The source code is free to download.
Functional requirements of Internship Portal Project in PHP
Administrator Side
- Add, delete, view, update and search Administrator Accounts
- Add, delete, view, update and search Internee Accounts
- Add, delete, view, update and search Job Opportunity List
- Add, delete, view, update and search Internee Particulars
Internee
- Login/Registration
- Browse/Explore Internship Job Opportunities
- Send Application
How to Run of Internship Portal Management System Project in PHP
Download Code-of-Internship-Portal-Project-PHP
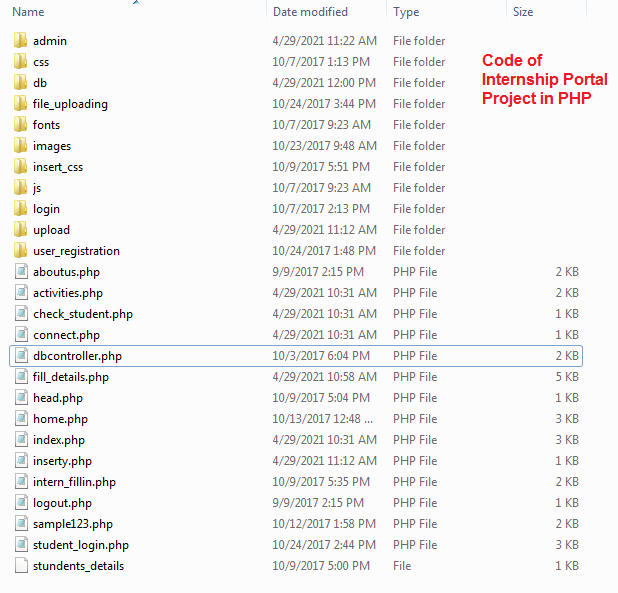
student_login.php
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 |
_<?php if (isset($_POST['submit'])) { $dbconn = mysqli_connect('localhost','root','','db_issm'); //include("config.php"); session_start(); $username=$_POST['user_name']; $password = $_POST["password"]; $_SESSION['user_name']=$username; $query = mysqli_query( $dbconn, "SELECT * FROM registered_users WHERE user_name='$username' and password='$password'"); if (mysqli_num_rows($query) != 0) { echo "<script language='javascript' type='text/javascript'> location.href='fill_details.php' </script>"; } else { echo "<script type='text/javascript'>alert('User Name Or Password Invalid!')</script>"; } } ?> <html> <head> <link rel="stylesheet" type="text/css" href="css/login.css"> <link rel="stylesheet" type="text/css" href="css/bootstrap.css"> <link rel="stylesheet" type="text/css" href="bootstrap/css/jquery.dataTables.css"> <link rel ="stylesheet" type="text/css" href ="css/bootstrap.min.css"> <script type="text/javascript" src="js/script1.js"></script> </head> <?php include("head.php");?> <div class="container"> <div class="row" id="pwd-container"> <div class="col-md-8"> <section class="login-form"> <form method="post" action="#" role="login"> <img src="img/icho.jpg" class="img-responsive" alt="" /> <input type="text" name="user_name" placeholder="enter username " required class="form-control input-lg" value="" /> <input type="password" name="password" class="form-control input-lg" id="password" placeholder="Password" required="" /> <div class="pwstrength_viewport_progress"></div> <button type="submit" name="submit" class="btn btn-lg btn-primary btn-block">Sign in</button> <div> <a href="index.php">Home </a> <a href="user_registration/index.php">Register </a> or <a href="#">reset password</a> </div> </form> </section> </div> <div class ="col-md-4"> </div> </div> </html> |
intern_fillin.php
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 |
<?php error_reporting(0); $reg_no=$_POST['reg_no']; $first_name =$_POST['first_name']; $last_name =$_POST['last_name']; $age=$_POST['age']; if (isset($_POST['insert_button'])){ {header('location:file_uploading/index.php'); $con=mysqli_connect("localhost","root","","db_issm"); // Check connection if (mysqli_connect_errno()) { echo "Failed to connect to MySQL: " . mysqli_connect_error(); } else{ $sql= "INSERT INTO students (reg_no, first_name, last_name,age) VALUES ('$reg_no', '$first_name', ' $last_name','$age')"; if (!mysqli_query($con,$sql)) { die('Error: ' . mysqli_error($con)); } else{ ?> <?php } } } mysqli_close($con); ?> <form name="insert" method="post" action=""> <input type="text" name="reg_no" placeholder="Registration Number" required> <br> <input type="text" name="first_name" placeholder="Full Name" required> <br> <input type="text" name="last_name" placeholder="Full Name" required> <br> <input type="text" name="age" placeholder="Age" required> <br> <input type="submit" name="insert_button" value="Insert Record" required> </form> |
inserty.php
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 |
<html> <body> <?php $con = mysqli_connect("localhost","root","","db_issm"); if (!$con) { die('Could not connect: ' . mysqli_error($con)); } mysqli_select_db($con, "db_issm"); $fname = time().'_'.$_FILES['file']['name']; $loc = './upload/'; $move = move_uploaded_file($_FILES['file']['tmp_name'], $loc.$fname); if(!$move) exit; // $fname = ''; $sql="INSERT INTO fill_details (company_name, first_name, last_name,email,gender,file) VALUES ( '$_POST[company_name]','$_POST[first_name]','$_POST[last_name]','$_POST[email]','$_POST[gender]','$fname')"; if (!mysqli_query($con,$sql)) { die('Error: ' . mysqli_error()); } header('location:file_uploading/index.php?success'); mysqli_close($con); ?> </body> </html> |
fill_details.php
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 |
<?php include 'head.php';?> <br> <br> <br> <br> <br> <?php if(!empty($_POST["insert_button"])) { /* Form Required Field Validation */ foreach($_POST as $key=>$value) { if(empty($_POST[$key])) { $error_message = "All Fields are required"; break; } } /* Email Validation */ if(!isset($error_message)) { if (!filter_var($_POST["email"], FILTER_VALIDATE_EMAIL)) { $error_message = "Invalid Email Address"; } } /* Validation to check if gender is selected */ if(!isset($error_message)) { if(!isset($_POST["gender"])) { $error_message = " All Fields are required"; } } /* Validation to check if Terms and Conditions are accepted */ if(!isset($error_message)) { if(!isset($_POST["terms"])) { $error_message = "Accept Terms and Conditions to Register"; } } } ?> <html> <head> <link rel="stylesheet" type="text/css" href="css/bootstrap.css"> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.2.1/jquery.min.js"></script> <script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/js/bootstrap.min.js"></script> <link rel = "stylesheet" type = "text/css" href = "css/jquery-ui.css" /> <link rel = "stylesheet" type = "text/css" href = "css/jquery.dataTables.css" /> <style> body{ width:610px; font-family:calibri; } .error-message { margin-top: 30px; padding: 50px 20px; background: #fff1f2; border: #ffd5da 1px solid; color: #d6001c; border-radius: 4px; } .success-message { padding: 7px 10px; background: #cae0c4; border: #c3d0b5 1px solid; color: #027506; border-radius: 4px; } .demo-table { background: white; width: 100%; border-spacing: initial; margin: 2px 0px; word-break: break-word; table-layout: auto; line-height: 1.8em; color: #333; border-radius: 4px; padding: 20px 40px; text-align: center; } .demo-table td { padding: 15px 0px; } .demoInputBox { padding: 10px 30px; border: #a9a9a9 1px solid; border-radius: 4px; } .btnRegister { padding: 10px 30px; background-color: #3367b2; border: 0; color: #FFF; cursor: pointer; border-radius: 4px; margin-left: 10px; } </style> </head> <body> <form name="frmRegistration" method="post" enctype="multipart/form-data" action="inserty.php"> <table border="0" width="500" align="center" class="demo-table"> <?php if(!empty($success_message)) { ?> <div class="success-message"><?php if(isset($success_message)) echo $success_message; ?></div> <?php } ?> <?php if(!empty($error_message)) { ?> <div class="error-message"><?php if(isset($error_message)) echo $error_message; ?></div> <?php } ?> <tr> <td>company_name</td> <td><input type="text" class="demoInputBox" name="company_name" minlength="3" maxlength="16" value=" <?php if(isset($_POST['company_name'])) echo $_POST['company_name']; ?>" required ></td> </tr> <tr> <td>first_name</td> <td><input type="text" class="demoInputBox" name="first_name"minlength="3" maxlength="16" value="<?php if(isset($_POST['first_name'])) echo $_POST['first_name']; ?>" required ></td> </tr> <tr> <td>Last Name</td> <td><input type="text" class="demoInputBox" name="last_name" minlength="3" maxlength="16" value="<?php if(isset($_POST['last_name'])) echo $_POST['last_name']; ?>" required ></td> </tr> <tr> <td>email</td> <td><input type="email" class="demoInputBox" name="email" value="<?php if(isset($_POST['email'])) echo $_POST['email']; ?>" required ></td></tr> <tr> <tr> <td>Gender</td> <td><input type="radio" name="gender" value="Male" <?php if(isset($_POST['gender']) && $_POST['gender']=="Male") { ?>checked<?php } ?> required > Male <input type="radio" name="gender" value="Female" <?php if(isset($_POST['gender']) && $_POST['gender']=="Female") { ?>checked<?php } ?> required > Female </td> </tr> <tr> <td>Upload cv:</td> <td> <input type="file" name="file" required /> </td> </tr> <tr> <td colspan=2> <input type="checkbox" name="terms"> I accept Terms and Conditions <input type="submit" name="insert_button" value="Apply" class="btnRegister" required ></td> </tr> </table> </body> </html> |
dbcontroller.php
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 |
<?php class DBController { private $host = "localhost"; private $user = "root"; private $password = ""; private $database = "db_issm"; private $conn; function __construct() { $this->conn = $this->connectDB(); } function connectDB() { $conn = mysqli_connect($this->host,$this->user,$this->password,$this->database); return $conn; } function runQuery($query) { $result = mysqli_query($this->conn,$query); while($row=mysqli_fetch_assoc($result)) { $resultset[] = $row; } if(!empty($resultset)) return $resultset; } function numRows($query) { $result = mysqli_query($this->conn,$query); $rowcount = mysqli_num_rows($result); return $rowcount; } function updateQuery($query) { $result = mysqli_query($this->conn,$query); if (!$result) { die('Invalid query: ' . mysql_error()); } else { return $result; } } function insertQuery($query) { $result = mysqli_query($this->conn,$query); if (!$result) { die('Invalid query: ' . mysql_error()); } else { return $result; } } function deleteQuery($query) { $result = mysqli_query($this->conn,$query); if (!$result) { die('Invalid query: ' . mysql_error()); } else { return $result; } } } ?> |